|
| QGridLayout () |
|
| QGridLayout (QWidget *parent) |
|
| ~QGridLayout () |
|
void | addItem (QLayoutItem *item, int row, int column, int rowSpan=1, int columnSpan=1, Qt::Alignment alignment=Qt::Alignment ()) |
|
void | addLayout (QLayout *layout, int row, int column, int rowSpan, int columnSpan, Qt::Alignment alignment=Qt::Alignment ()) |
|
void | addLayout (QLayout *layout, int row, int column, Qt::Alignment alignment=Qt::Alignment ()) |
|
void | addWidget (QWidget *widget, int row, int column, int rowSpan, int columnSpan, Qt::Alignment alignment=Qt::Alignment ()) |
|
void | addWidget (QWidget *widget, int row, int column, Qt::Alignment alignment=Qt::Alignment ()) |
|
QRect | cellRect (int row, int column) const |
|
int | columnCount () const |
|
int | columnMinimumWidth (int column) const |
|
int | columnStretch (int column) const |
|
int | count () const override |
|
Qt::Orientations | expandingDirections () const override |
|
void | getItemPosition (int index, int *row, int *column, int *rowSpan, int *columnSpan) const |
|
bool | hasHeightForWidth () const override |
|
int | heightForWidth (int width) const override |
|
int | horizontalSpacing () const |
|
void | invalidate () override |
|
QLayoutItem * | itemAt (int index) const override |
|
QLayoutItem * | itemAtPosition (int row, int column) const |
|
QSize | maximumSize () const override |
|
int | minimumHeightForWidth (int width) const override |
|
QSize | minimumSize () const override |
|
Qt::Corner | originCorner () const |
|
int | rowCount () const |
|
int | rowMinimumHeight (int row) const |
|
int | rowStretch (int row) const |
|
void | setColumnMinimumWidth (int column, int minSize) |
|
void | setColumnStretch (int column, int stretch) |
|
void | setGeometry (const QRect &rect) override |
|
void | setHorizontalSpacing (int spacing) |
|
void | setOriginCorner (Qt::Corner corner) |
|
void | setRowMinimumHeight (int row, int minSize) |
|
void | setRowStretch (int row, int stretch) |
|
void | setSpacing (int spacing) |
|
void | setVerticalSpacing (int spacing) |
|
QSize | sizeHint () const override |
|
int | spacing () const |
|
QLayoutItem * | takeAt (int index) override |
|
int | verticalSpacing () const |
|
| QLayout () |
|
| QLayout (QWidget *parent) |
|
bool | activate () |
|
void | addWidget (QWidget *w) |
|
QMargins | contentsMargins () const |
|
QRect | contentsRect () const |
|
QSizePolicy::ControlTypes | controlTypes () const override |
|
Qt::Orientations | expandingDirections () const override |
|
QRect | geometry () const override |
|
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
|
virtual int | indexOf (QWidget *widget) const |
|
void | invalidate () override |
|
bool | isEmpty () const override |
|
bool | isEnabled () const |
|
QLayout * | layout () override |
|
int | margin () const |
|
QSize | maximumSize () const override |
|
QWidget * | menuBar () const |
|
QSize | minimumSize () const override |
|
QWidget * | parentWidget () const |
|
void | removeItem (QLayoutItem *item) |
|
void | removeWidget (QWidget *widget) |
|
virtual QLayoutItem * | replaceWidget (QWidget *from, QWidget *to, Qt::FindChildOptions options=Qt::FindChildrenRecursively) |
|
bool | setAlignment (QLayout *layout, Qt::Alignment alignment) |
|
void | setAlignment (Qt::Alignment alignment) |
|
bool | setAlignment (QWidget *widget, Qt::Alignment alignment) |
|
void | setContentsMargins (const QMargins &margins) |
|
void | setContentsMargins (int left, int top, int right, int bottom) |
|
void | setEnabled (bool enable) |
|
void | setGeometry (const QRect &rect) override |
|
void | setMargin (int margin) |
|
void | setMenuBar (QWidget *widget) |
|
void | setSizeConstraint (SizeConstraint constraint) |
|
void | setSpacing (int spacing) |
|
SizeConstraint | sizeConstraint () const |
|
int | spacing () const |
|
void | update () |
|
| QObject (QObject *parent=nullptr) |
|
| ~QObject () |
|
bool | blockSignals (bool block) |
|
const QList< QObject * > & | children () const |
|
bool | connect (const QObject *sender, const QString &signalMethod, const QString &location, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
bool | connect (const QObject *sender, const QString &signalMethod, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
bool | disconnect (const QObject *receiver, const QString &slotMethod=QString ()) const |
|
bool | disconnect (const QString &signalMethod, const QString &location, const QObject *receiver=nullptr, const QString &slotMethod=QString ()) const |
|
bool | disconnect (const QString &signalMethod=QString (), const QObject *receiver=nullptr, const QString &slotMethod=QString ()) const |
|
void | dumpObjectInfo () |
|
void | dumpObjectTree () |
|
QList< QString > | dynamicPropertyNames () const |
|
virtual bool | event (QEvent *event) |
|
virtual bool | eventFilter (QObject *watched, QEvent *event) |
|
template<typename T > |
T | findChild (const QString &childName=QString ()) const |
|
template<class T > |
QList< T > | findChildren (const QRegularExpression ®Exp, Qt::FindChildOptions options=Qt::FindChildrenRecursively) const |
|
template<class T > |
QList< T > | findChildren (const QString &childName=QString (), Qt::FindChildOptions options=Qt::FindChildrenRecursively) const |
|
bool | inherits (const QString &className) const |
|
void | installEventFilter (QObject *filterObj) |
|
bool | isWidgetType () const |
|
bool | isWindowType () const |
|
void | killTimer (int id) |
|
const QMetaObject * | metaObject () const |
|
void | moveToThread (QThread *targetThread) |
|
QString | objectName () const |
|
QObject * | parent () const |
|
template<class T = QVariant> |
T | property (const QString &name) const |
|
void | removeEventFilter (QObject *obj) |
|
void | setObjectName (const QString &name) |
|
void | setParent (QObject *parent) |
|
bool | setProperty (const QString &name, const QVariant &value) |
|
bool | signalsBlocked () const |
|
int | startTimer (int interval, Qt::TimerType timerType=Qt::CoarseTimer) |
|
QThread * | thread () const |
|
| QLayoutItem (Qt::Alignment alignment=Qt::Alignment ()) |
|
virtual | ~QLayoutItem () |
|
Qt::Alignment | alignment () const |
|
void | setAlignment (Qt::Alignment alignment) |
|
virtual QSpacerItem * | spacerItem () |
|
virtual QWidget * | widget () |
|
|
enum | SizeConstraint |
|
void | destroyed (QObject *obj=nullptr) |
|
void | objectNameChanged (const QString &objectName) |
|
void | deleteLater () |
|
static QSize | closestAcceptableSize (const QWidget *widget, const QSize &size) |
|
static bool | connect (const QObject *sender, const QMetaMethod &signalMethod, const QObject *receiver, const QMetaMethod &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
static bool | connect (const QObject *sender, const QString &signalMethod, const QObject *receiver, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection, const QString &location=QString ()) |
|
static bool | connect (const QObject *sender, const QString &signalMethod, const QString &location, const QObject *receiver, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class SlotClass , class... SlotArgs, class SlotReturn > |
static bool | connect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, SlotReturn (SlotClass::*slotMethod)(SlotArgs...), Qt::ConnectionType type=Qt::AutoConnection) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class T > |
static bool | connect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, T slotLambda, Qt::ConnectionType type=Qt::AutoConnection) |
|
static bool | disconnect (const QObject *sender, const QMetaMethod &signalMethod, const QObject *receiver, const QMetaMethod &slotMethod) |
|
static bool | disconnect (const QObject *sender, const QString &signalMethod, const QObject *receiver, const QString &slotMethod) |
|
static bool | disconnect (const QObject *sender, const QString &signalMethod, const QString &location, const QObject *receiver, const QString &slotMethod) |
|
static bool | disconnect (const QObject *sender, std::nullptr_t, const QObject *receiver, std::nullptr_t) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class SlotClass , class... SlotArgs, class SlotReturn > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, SlotReturn (SlotClass::*slotMethod)(SlotArgs...)) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, std::nullptr_t slotMethod=nullptr) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class T > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, T slotMethod) |
|
static QMetaObject & | staticMetaObject () |
|
static QString | tr (const char *text, const char *comment=nullptr, std::optional< int > numArg=std::optional< int >()) |
|
T | qobject_cast (QObject *object) |
|
| QObjectList |
|
The QGridLayout class arranges child widgets in a grid. This class controls how the available space from the parent layout or parent widget is used.
Each managed widget or layout is normally added to a cell of its own using addWidget(). A widget can be configured to occupy multiple cells using the row and column spanning overloads of addItem() and addWidget(). If you do this QGridLayout will guess how to distribute the size over the columns/rows based on the stretch factors.
Each column has a minimum width and a stretch factor. The minimum width is the larger value between setColumnMinimumWidth() and the minimum width of each widget in that column. The stretch factor is set using setColumnStretch() and determines how much of the available space the column will get over and above its necessary minimum.
To remove a widget from a layout call removeWidget(). Calling QWidget::hide() on a widget also effectively removes the widget from the layout until QWidget::show() is called.
Example
The image shown below is a section of a dialog with five columns and three rows in the grid. Each row and column is outlined in magenta.
Columns 0, 2 and 4 in this dialog consist of a QLabel, QLineEdit, and a QListWidget. Columns 1 and 3 are placeholders created with setColumnMinimumWidth(). Row 0 consists of three QLabel objects, row 1 of three QLineEdit objects and row 2 of three QListWidget objects. There are placeholder columns (1 and 3) to get the right amount of space between the columns.
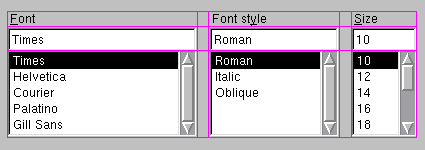
The columns and rows are not equal in height and width. If you want two columns to have the same width you must set their minimum widths and stretch factors to be the same. Do this by using setColumnMinimumWidth() and setColumnStretch().
If the QGridLayout is not the top-level layout (i.e. does not manage all of the widget's area and children), you must add it to its parent layout when you create it, but before you do anything with it. The normal way to add a layout is by calling addLayout() on the parent layout.
Once you have added your layout you can start putting widgets and other layouts into the cells of your grid layout using addWidget(), addItem(), and addLayout().
Margins
QGridLayout also includes two margin widths: the contents margin and the spacing(). The contents margin is the width of the reserved space along each of the QGridLayout's four sides. The spacing() is the width of the automatically allocated spacing between neighboring boxes.
The default contents margin values are provided by the style. The default value CopperSpice styles specify is 9 for child widgets and 11 for windows. The spacing defaults to the same as the margin width for a top-level layout, or to the same as the parent layout.
- See also
- QBoxLayout, QStackedLayout, Layout Management