The QSystemTrayIcon class provides an icon for an application in the system tray.
More...
|
| QSystemTrayIcon (const QIcon &icon, QObject *parent=nullptr) |
|
| QSystemTrayIcon (QObject *parent=nullptr) |
|
| ~QSystemTrayIcon () |
|
QMenu * | contextMenu () const |
|
QRect | geometry () const |
|
QIcon | icon () const |
|
bool | isVisible () const |
|
void | setContextMenu (QMenu *menu) |
|
void | setIcon (const QIcon &icon) |
|
void | setToolTip (const QString &tip) |
|
QString | toolTip () const |
|
| QObject (QObject *parent=nullptr) |
|
| ~QObject () |
|
bool | blockSignals (bool block) |
|
const QList< QObject * > & | children () const |
|
bool | connect (const QObject *sender, const QString &signalMethod, const QString &location, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
bool | connect (const QObject *sender, const QString &signalMethod, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
bool | disconnect (const QObject *receiver, const QString &slotMethod=QString ()) const |
|
bool | disconnect (const QString &signalMethod, const QString &location, const QObject *receiver=nullptr, const QString &slotMethod=QString ()) const |
|
bool | disconnect (const QString &signalMethod=QString (), const QObject *receiver=nullptr, const QString &slotMethod=QString ()) const |
|
void | dumpObjectInfo () |
|
void | dumpObjectTree () |
|
QList< QString > | dynamicPropertyNames () const |
|
virtual bool | eventFilter (QObject *watched, QEvent *event) |
|
template<typename T > |
T | findChild (const QString &childName=QString ()) const |
|
template<class T > |
QList< T > | findChildren (const QRegularExpression ®Exp, Qt::FindChildOptions options=Qt::FindChildrenRecursively) const |
|
template<class T > |
QList< T > | findChildren (const QString &childName=QString (), Qt::FindChildOptions options=Qt::FindChildrenRecursively) const |
|
bool | inherits (const QString &className) const |
|
void | installEventFilter (QObject *filterObj) |
|
bool | isWidgetType () const |
|
bool | isWindowType () const |
|
void | killTimer (int id) |
|
const QMetaObject * | metaObject () const |
|
void | moveToThread (QThread *targetThread) |
|
QString | objectName () const |
|
QObject * | parent () const |
|
template<class T = QVariant> |
T | property (const QString &name) const |
|
void | removeEventFilter (QObject *obj) |
|
void | setObjectName (const QString &name) |
|
void | setParent (QObject *parent) |
|
bool | setProperty (const QString &name, const QVariant &value) |
|
bool | signalsBlocked () const |
|
int | startTimer (int interval, Qt::TimerType timerType=Qt::CoarseTimer) |
|
QThread * | thread () const |
|
|
static bool | isSystemTrayAvailable () |
|
static bool | supportsMessages () |
|
static bool | connect (const QObject *sender, const QMetaMethod &signalMethod, const QObject *receiver, const QMetaMethod &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
static bool | connect (const QObject *sender, const QString &signalMethod, const QObject *receiver, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection, const QString &location=QString ()) |
|
static bool | connect (const QObject *sender, const QString &signalMethod, const QString &location, const QObject *receiver, const QString &slotMethod, Qt::ConnectionType type=Qt::AutoConnection) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class SlotClass , class... SlotArgs, class SlotReturn > |
static bool | connect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, SlotReturn (SlotClass::*slotMethod)(SlotArgs...), Qt::ConnectionType type=Qt::AutoConnection) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class T > |
static bool | connect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, T slotLambda, Qt::ConnectionType type=Qt::AutoConnection) |
|
static bool | disconnect (const QObject *sender, const QMetaMethod &signalMethod, const QObject *receiver, const QMetaMethod &slotMethod) |
|
static bool | disconnect (const QObject *sender, const QString &signalMethod, const QObject *receiver, const QString &slotMethod) |
|
static bool | disconnect (const QObject *sender, const QString &signalMethod, const QString &location, const QObject *receiver, const QString &slotMethod) |
|
static bool | disconnect (const QObject *sender, std::nullptr_t, const QObject *receiver, std::nullptr_t) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class SlotClass , class... SlotArgs, class SlotReturn > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, SlotReturn (SlotClass::*slotMethod)(SlotArgs...)) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, std::nullptr_t slotMethod=nullptr) |
|
template<class Sender , class SignalClass , class... SignalArgs, class Receiver , class T > |
static bool | disconnect (const Sender *sender, void (SignalClass::*signalMethod)(SignalArgs...), const Receiver *receiver, T slotMethod) |
|
static QMetaObject & | staticMetaObject () |
|
static QString | tr (const char *text, const char *comment=nullptr, std::optional< int > numArg=std::optional< int >()) |
|
The QSystemTrayIcon class provides an icon for an application in the system tray. Modern operating systems usually provide a special area on the desktop, called the system tray or notification area, where long-running applications can display icons and short messages. The activated() signal is emitted when the user activates the icon.
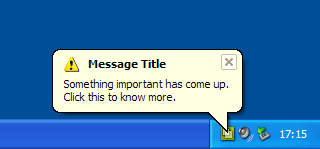
The QSystemTrayIcon class can be used on the following platforms.
-
All supported versions of Windows
-
All window managers for X11 which implement the freedesktop system tray specification, including recent versions of KDE and GNOME.
-
All supported versions of Mac OS X. Note that the Growl notification system must be installed for QSystemTrayIcon::showMessage() to display messages.
To check whether a system tray is present on the user's desktop, call the QSystemTrayIcon::isSystemTrayAvailable() static function. To add a system tray entry, create a QSystemTrayIcon object, call setContextMenu() to provide a context menu for the icon, and call show() to make it visible in the system tray. Status notification messages can be displayed at any time using showMessage().
If the system tray is unavailable when a system tray icon is constructed, but becomes available later, QSystemTrayIcon will automatically add an entry for the application in the system tray if the icon is visible.
Only on X11, when a tooltip is requested, the QSystemTrayIcon receives a QHelpEvent of type QEvent::ToolTip. Additionally, the QSystemTrayIcon receives wheel events of type QEvent::Wheel. These are not supported on any other platform.
- See also
- QDesktopServices, QDesktopWidget
Shows a balloon message for the entry with the given title, msg, and icon for the time specified in msecs. The title and msg must be plain text strings.
When the balloon message is clicked the messageClicked() signal will emitted.
The display of messages are dependent on the system configuration and user preferences and in some cases messages may not appear at all. The system tray should not be relied upon as the sole means for providing critical information. On Windows, the msecs is usually ignored by the system when the application has focus. On OS X, the Growl notification system must be installed for this method to display messages.
- See also
- show(), supportsMessages()